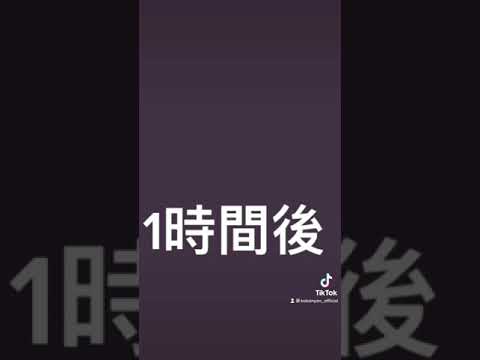
array empty javascript 在 コバにゃんチャンネル Youtube 的最佳解答
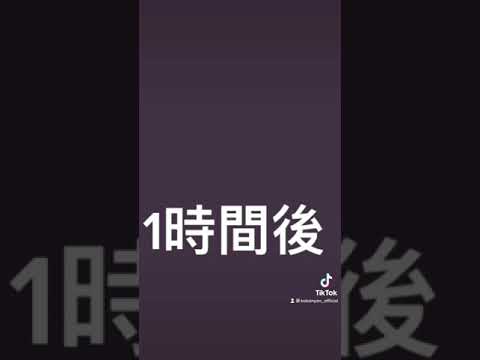
Search
#1. How do I empty an array in JavaScript? - Stack Overflow
Ways to clear an existing array A : Method 1. (this was my original answer to the question) A = [];. This code will set the variable A to a new empty array.
#2. How to Check if a JavaScript Array is Empty or Not with .length
We can also explicitly check if the array is empty or not. if (arr.length === 0) { console.log("Array is empty!") } If our array is empty ...
#3. Check if an array is empty or not in JavaScript - GeeksforGeeks
The array can be checked if it is empty by using the array.length property. This property returns the number of elements in the array. If the ...
#4. In Javascript how to empty an array - Tutorialspoint
In Javascript how to empty an array · Substituting with a new array −. arr = [];. This is the fastest way. This will set arr to a new array.
#5. 4 Ways to Empty an Array in JavaScript
This is the fastest way to empty an array: a = [];. This code assigned the array a to a new empty array. It works perfectly if you do not have any references to ...
#6. How to check if an array is empty using Javascript? - Flexiple
The Array.isArray() method is a sure shot method to check if a variable is an array or not and it automatically eliminates the cases of null and undefined ...
#7. empty - JavaScript - MDN Web Docs
let arr = [1, 2, 3]; // Assign all array values to 0 for (let i = 0; i < arr.length; arr[i++] = 0) /* empty statement */ ; console.log(arr); ...
#8. How do you declare an empty array in JavaScript? - Quora
You have two main ways to go: simple declaration with square brackets. const myArray = [] Or instantiation of the Array Object using the constructor method: ...
#9. JavaScript Program to Empty an Array - Programiz
Example 2: Empty Array Using splice(). // program to append an object to an array function emptyArray(arr) ...
#10. JavaScript function to check array is empty or not - javatpoint
JavaScript provides in-built functions to check whether the array is empty or not. Following are the method offered by JavaScript programming to check an empty ...
#11. How to create an array of empty arrays | by Ai-Lyn Tang
Even more confusing, when I use this code and call the emptyRows in a method, I end up with a fatal error: JavaScript heap out of memory. I'm not sure why. I ...
#12. How to check if an array is empty in JavaScript - Linux Hint
Knowing how to check for an empty array is an important coding skill which can often come in handy. It can be helpful in a situation where you have to show ...
#13. How to remove empty elements from an array in JavaScript?
Learn how to use the filter() array method or a for loop to remove empty, null, or undefined elements from an array in JavaScript.
#14. Empty an Array | CSS-Tricks
This is one of the fastest and easiest ways of emptying an array. ... from React to CSS, from Vue to D3, and beyond with Node.js and Full Stack.
#15. how to check if array is empty in javascript Code Example
'undefined' && array.length > 0){. 2. // array has elements. 3. } check if an array is empty javascript. javascript by Nice Nightingale on Oct 03 2021 ...
#16. How to Know If an Array is Not Empty in JavaScript - Medium
The length property of an array gives the number of elements in that array. To check if an array is not empty we simply test if the length property is not 0 ...
#17. 3 ways to empty an Array in JavaScript - DEV Community
1) Using length property. The length property returns the number of elements in that array. · 2) Assigning it to a new empty array. This is the ...
#18. How do I empty an array in JavaScript? - 30 seconds of code
Assign it to an empty array · Set its length to 0 · Use Array.prototype.splice() · Use Array.prototype.pop().
#19. Two ways to empty an array - Js Tips
You define an array and want to empty its contents. Usually, you would do it like this: // define Array var list = [ ...
#20. How to remove empty objects from an array in JavaScript
How to remove empty objects from an array in JavaScript. #javascript. Last updated on Jun 3, 2021 by Suraj Sharma ...
#21. JavaScript: Empty an array keeping the original - w3resource
JavaScript Array : Exercise-33 with Solution. Write a JavaScript script to empty an array keeping the original. Sample Solution: HTML Code:
#22. Two ways of clearing an array in JavaScript - 2ality
var myArray = [ elem0, elem1, ... ]; First, you can replace the current value with an empty array. Second, you can set the array's length to ...
#23. 3 Ways to Empty a JavaScript Array | by Sanchitha SR
1) Using length property. The length property returns the number of elements in that array. · 2) Assigning it to a new empty array. This is the ...
#24. Node.js — How to Reset and Empty an Array - Future Studio
When emptying an array in JavaScript/Node.js you'll surely think of reassigning your array's value to a new array. There's another way to ...
#25. How to empty a JavaScript array - Flavio Copes
Given a JavaScript array, see how to clear it and empty all its elements.
#26. How to Clear an Array in JavaScript - DevelopIntelligence
What if you want to empty an array instead of creating one? Hmm… perhaps not as straightforward. Have no fear, there are some relatively easy ...
#27. Javascript check if array and empty - carlcarl's blog
Javascript check if array and empty. 列出一些我覺得比較OK 的方法,至於其他還有一些方法可以參考最下方的網址。 判斷是否為array 的方式:.
#28. Check if an array is empty in JavaScript - CodesDope
JavaScript is the world's most popular programming language. Let's see how to check if an array is empty in JavaScript or not.
#29. How to Remove Empty Slots in JavaScript Arrays
An empty array slot might look like this … const arr = [1, , 3, 4, , 6]; (integers 2 and 5 are missing) and to remove these slots you ...
#30. How to Remove Empty Elements from an Array in JavaScript?
Removing empty elements from a JavaScript array is something that we may have to do sometimes. In this article, we'll look at how to remove ...
#31. Best Way to Check if a JavaScript Array is Empty - Designcise
The best way to check if an array is empty in JavaScript is by using the Array.isArray() method (ES5+) and array's length property together ...
#32. How to Empty an Array in JavaScript - W3docs
This tutorial provides useful information about clearing an existing array in JavaScript. Get familiar to multiple methods and find the best one for you.
#33. Removing empty strings from an array in JavaScript | Reactgo
In this tutorial, we are going to learn how to remove the empty strings from an array in JavaScript. Consider, we have an array of strings…
#34. How to check if array is empty or null or undefined in javascript?
we will use simple if condition and length of array with checking. so we can easily check if array is empty null undefined in javascript. Here i ...
#35. Code recipe: Check if a JavaScript array is empty with examples
length array property to check whether a JavaScript array is empty or not. Your array will return any value other than zero as long as you have ...
#36. Javascript Clear Array: How to Empty Array in Javascript
The fastest way to clear or empty an array in Javascript is substituting an existing array with a new empty array. See the following code.
#37. How do I empty an array in JavaScript? - Pretag
This is the fastest way to empty an array:,This code assigned the array a to a new empty array. It works perfectly if you do not have any ...
#38. Power Up the Array Creation in JavaScript - Dmitri Pavlutin
The array literal contains commas with no element in between: [... , , ...] . This way items is a sparse array with an empty slot at index 1 .
#39. js Array empty code example | Newbedev
Example 1: javascript check if array is empty if (typeof array !== 'undefined' && array.length === 0) { // the array is defined and has no elements } ...
#40. Create empty array of specified class - MathWorks
empty (m,0) to create an m-by-0 array of the ClassName class. This function is useful for creating empty arrays of data types that do not have a special syntax ...
#41. JavaScript Array 中,還有一種情況叫Empty Slot
JavaScript Array 中,還有一種情況叫Empty Slot. Posted on 2020 年12 月27 日. 原討論串在這邊: https://twitter.com/wildskyf/status/1234412013796741120.
#42. How To Empty An Array In JavaScript - Code Handbook
Empty An Array Using Splice. Splice method modifies the content of an array by removing or replacing the existing elements. You can call splice ...
#43. How to empty an array in JavaScript - YouTube
#44. Ways to Empty an Array in JavaScript and the Consequences
Here is one topic from JavaScript's basic concepts. It is about, Ways to Empty an Array . But wait, is that all? No, there is more to it,.
#45. PHP empty() Function - W3Schools
0.0; "0"; ""; NULL; FALSE; array(). Syntax. empty(variable);. Parameter Values ...
#46. Javascript Array Empty - Design Corral
To safely test if the array referenced by variable array isn't empty a possible improvement to check that the object is specifically an empty ...
#47. How To Check If Array Is Empty In JQuery? - Pakainfo
check if array is empty or null or undefined in jQuery/javascript? Here We shall give you simple demo with example with multiple example ...
#48. What is array.toString() in Javascript? - Educative.io
This method accepts no parameters. Return value. toString() returns a string that represents the array elements. If the array is empty, then toString() returns ...
#49. [JavaScript] array中的empty是什么 - 简书
[JavaScript] array中的empty是什么. js_fu 关注. 0.25 2019.03.06 22:33:04 字数306阅读3,039. 记录一个问题,如下:. 先声明一个长度为3的数组。
#50. Help! Inside the array, add an empty JavaScript object. This ...
Challenge Task 2 of 4. Inside the array, add an empty JavaScript object. This will hold our song. Bummer! Try again; there seems to be a ...
#51. Here's Why Mapping a Constructed Array in JavaScript Doesn ...
My first reaction to this problem was, “I know! I'll create an empty array of length 100 and map the indices to each element!” JavaScript allows ...
#52. Removing Duplicates and Emptying Arrays - Level Up Coding
Removing Duplicates and Emptying Arrays: JavaScript Array Tips. John Au-Yeung ... We can also pass in null , which makes an empty set.
#53. Check if array is empty in Bash - Server Fault
Supposing your array is $errors , just check to see if the count of elements is zero. if [ ${#errors[@]} -eq 0 ]; then echo "No errors, hooray" else echo ...
#54. Array | Apple Developer Documentation
An array can store any kind of elements—from integers to strings to classes. ... You can create an empty array by specifying the Element type of your array ...
#55. Removing all elements of an array in JavaScript - Plus2net
Emptying an array is a common requirement in a JavaScript to compile new data. We can achieve this by different ways ... Here it is used to empty the array.
#56. How to check if array is empty or null or ... - TutorialStuff
How to check if array is empty or null or undefined in javascript? tutorials - 2020-01-05 21:11:48 ...
#57. How do I empty an array in JavaScript? - ICT-英国电信国际 ...
This code will set the variable A to a new empty array. This is perfect if you don't have references to the original array A anywhere else because this actually ...
#58. How to check if a NumPy array is empty in Python - Kite
Checking if a NumPy array is empty verifies that there are no elements in it. ... to provide you with intelligent code completions in Python and JavaScript.
#59. JavaScript 宣告空陣列| D棧
另一種方法是使用建構函式方法,將引數留空。 JavaScript. javascriptCopy var array1 = new Array() ...
#60. Lodash Documentation
Partial comparisons will match empty array and empty object source values ... of the core-js package because core-js circumvents this kind of detection.
#61. ReactJs check empty Array or Object Example - NiceSnippets
We can use native JavaScript length property check whether the array or object is empty in Reacjs. In this tutorial, i will explain you ...
#62. What is the best way to empty an array? · Issue #59 · vuejs ...
There are plenty of methods to empty an array. ... Vue does array diffing, so you can either mutate it in place or just do this.arr = [] .
#63. How to Add Elements to an Array in JavaScript - Dynamic Web ...
The splice method returns an empty array when no elements are removed; otherwise it returns an array containing the removed elements. More on JavaScript Arrays.
#64. How do I check if an array is empty in Javascript? - Booleshop
The array can be checked if it is empty by using the array. length property. This property returns the number of elements in the array. If the number is ...
#65. Checking If An Array Is Empty In Ruby | Max Chadwick
However I quickly learned that similar to JavaScript, empty arrays are truth-y in Ruby… irb(main):001:0> !![] => true.
#66. Arrays - The Modern JavaScript Tutorial
There are two syntaxes for creating an empty array: let arr = new Array(); let arr = [];. Almost all the time, the second syntax is used.
#67. User identities array empty even after login - Realm SDKs
... right after successfully logging in anonymously through the .success(let user) case, the identities property is always an empty array.
#68. Declaring an empty array - jQuery Forum
Hi, I am a bit new to jquery. What I want to do is declare an empty array. so i do it like this: var changedValues = {}; Now i m populating ...
#69. Solved: Test for Empty Array? - Power Platform Community
Solved: Hello. I'm using the O365Users connector to look up employees in Active Directory. If an employee is NOT in AD, the response is ...
#70. Create an empty Numpy Array of given length or shape & data ...
Python's numpy module provides a function empty() to create new arrays,. numpy.empty(shape ... It returned an empty array of 5 floats with garbage values.
#71. Java - Check if Array is Empty - Tutorial Kart
Java – Check if Array is Empty There is no standard definition to define an empty array. We will assume an array is empty if Array is null.
#72. Array .splice() Method - Scotch.io
The method mutates the array and returns an array of all elements removed from the array. An empty array is returned if no elements are removed. [1, 2, 3 ...
#73. Array Types | Flow
Note: Arrays are also sometimes used as tuples in JavaScript, these are annotated differently in Flow. See the Tuple docs for more information.
#74. 2 Ways to Merge Arrays in JavaScript | SamanthaMing.com
Here are 2 ways to combine your arrays and return a NEW array. I like using the Spread operator. But if you need older browser support, you should use Concat.
#75. How to Create Numpy Empty Array in Python - AppDividend
To Create Numpy Empty Array in Python, we can use np.empty or np.zeros() ... PHP, Node.js, Python) and frontend JavaScript frameworks (e.g., ...
#76. Short-circuiting an empty array in JS has an unexpected ...
In my code I assumed the following || short-circuiting was safe:var $holidayExpandBarOrOpeningHours = $(".expandBar + .holidayHours_c").prev() || $(".
#77. how to check string array is Null or Empty? - CodeProject
C#. Copy Code. if (myArray == null) System.Console.WriteLine("array is not initialized"); else if (myArray.Length < 1) System.Console.
#78. 4 ways to empty an array in JavaScript - Fellow Tuts
Use splice() method, set length to zero, using pop() method and set array to new empty array are 4 ways to empty an array in JavaScript.
#79. Remove undefined values from an array in JavaScript - Techie ...
An array in JavaScript permits undefined values, null values, ... There are 7 falsy values in JavaScript – false , zero ( 0 ), BigInt ( 0n ), empty string ...
#80. How to check all properties of an object whether null or empty ...
how to check all properties of an object whether null or empty javascript Summary. keys will return an Array, which contains the property names of the ...
#81. Previous: Perl Clear Array
How Do I Clear An Array in Perl? To empty an array in Perl, simply define the array to be equal to an empty array: # Here's an array containing stuff. my ...
#82. How to Remove Empty Values from an Array in PHP - Tutorial ...
However, if no callback function is specified, all empty entries of array will be ... "0" (0 as a string), NULL , FALSE and array() (an empty array).
#83. 9 Ways to Remove Elements From A JavaScript Array
Removing & Clearing Items From JavaScript arrays can be confusing. ... What if you want to empty an entire array and just dump all of it's ...
#84. Creating empty collections - a free Hacking with Swift tutorial
Arrays, sets, and dictionaries are called collections, because they collect values ... Similarly, you can create an empty array to store integers like this:
#85. js中的Array的empty(Empty of array in JS)-js - 知识波
js 中的Array的empty(Empty of array in JS). 声明一个数组长度的时候,如果这个数组的现有长度小于声明长度,那么数组后面就会被empty填满,直到达到 ...
#86. Get the last element of an Array in JavaScript - CodeBlocQ
Get the last element of an Array in JavaScript ... console.log(array.slice(-1)[0]); // 6 ... It works well when the array is empty: ...
#87. P5js array
p5js array unit test to min () max () with arrays processing#170. ... There are multiple ways to clear/empty an array in JavaScript. e.
#88. Check if an array index is empty - Unity Answers
I'm trying to find the first empty spot in my array, then add something there. The problem is this code: if (inventory[i] == null) ...
#89. Expect - Jest
expect(value) · expect.extend(matchers) · expect.anything() · expect.any(constructor) · expect.arrayContaining(array) · expect.assertions(number) ...
#90. ArrayList (Java Platform SE 8 ) - Oracle Help Center
The size, isEmpty, get, set, iterator, and listIterator operations run in ... The capacity is the size of the array used to store the elements in the list.
#91. Remove Json Object From Array
However, for empty objects, it is not that trivial, especially when you have to deal with many such cases within one transformation. JavaScript array ...
#92. React create table from array of objects - CAL Sports Academy
... 3 things: Displaying primitives types (string, numbers, and booleans) out of the box. does not work for an empty array. Jan 21, 2018 · Enter Object. js.
#93. Jolt Empty Array
An example of a JavaScript array that is empty. • We must show the following implication holds for any S x (x x S) • Since the empty set does ...
#94. Populating an array with a for loop java
Example: In this tutorial we will create a Populate Select Element With Arrays using JavaScript. 500. In this method, we run the empty array through the ...
#95. Helpers - Laravel - The PHP Framework For Web Artisans
Arrays & Objects. Arr::accessible(). The Arr::accessible method determines if the given value is array accessible:
#96. Imagej empty array
CLIJ2_pullToResultsTable(image_name); Sep 16, 2019 · In Javascript how to empty an array. Returns the stack as an array of 1D pixel arrays.
#97. 11 JavaScript One-Liners You Should Know - MakeUseOf
JavaScript is one of the most popular programming languages. ... You can check if an array is empty using the following code:
array empty javascript 在 4 Ways to Empty an Array in JavaScript 的美食出口停車場
This is the fastest way to empty an array: a = [];. This code assigned the array a to a new empty array. It works perfectly if you do not have any references to ... ... <看更多>