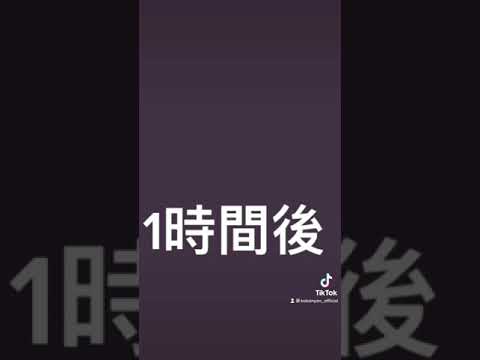
python serial write byte 在 コバにゃんチャンネル Youtube 的精選貼文
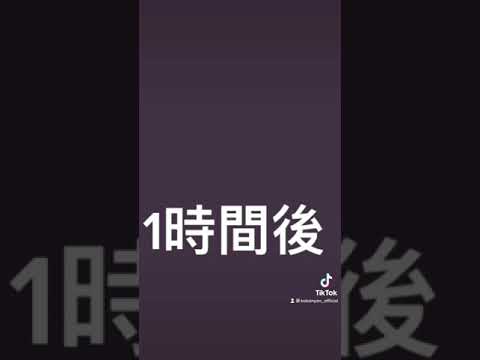
Search
#1. How can I send a byte array to a serial port using Python?
Try to declare the values explicitly as a bytearray and then write it to the serial port: import serial ser = serial.Serial('/dev/ttyACM0' ...
#2. pySerial API
Write the bytes data to the port. This should be of type bytes (or compatible such as bytearray or memoryview ). Unicode strings must be encoded (e.g. ...
#3. 利用python 經由serial port 輸出資料 - 給自己的學習記錄
Python 需安裝Python Serial Port Extension https://pypi.python.org/pypi/pyserial 安裝方式: ... ser.write(ary) time.sleep(0.1) ser.close()
#4. Byte manipulation on Python platform - JimmyIoT
This blog is to share some demo or guideline how to work the embedded byte operation on python. For example, if you are using the PYSERIAL ...
#5. Python serial.write方法代碼示例- 純淨天空
# 需要導入模塊: import serial [as 別名] # 或者: from serial import write [as 別名] def __checkType__(self, serial): byte = serial.read().decode("utf-8") print( ...
#6. Serial — python-periphery 1.1.0 documentation
... no flow control serial = Serial("/dev/ttyUSB0", 115200) serial.write(b"Hello World!") # Read up to 128 bytes with 500ms timeout buf = serial.read(128, ...
#7. Send bytes via serial port - Raspberry Pi Forums
Serial.write(byte(0x01)); With this sequence the roomba spins in counter clockwise direction. When I try to do the same in python (with the ...
#8. How to send bytes from pyserial to Arduino : r/learnpython
My sending code from Pyserial to Arduino is this: ... ser.write(header) for i in hexdata: ser.write(i.encode()) ser.write(trailer) ...
#9. Bytes and Unicode Strings - Problem Solving with Python
Before using PySerial to communicate with external hardware over the serial interface, it is import to understand the difference between bytes and unicode ...
#10. python 串口pyserial - CSDN博客
1、读取和发送的是byte形式,. 2、write和readline的内容以'\n'结尾. import sys import serial import serial.tools.list_ports
#11. Is arduino not recieving my byte sent - Programming Questions
Blackfin March 26, 2021, 3:15am #4. If you change the serial write in your Python code from: ser.write(bytes("b", 'utf-8')).
#12. How do you send multiple data bytes to Irobot Roomba via ...
I'm not sure if/how pyserial works with a bytearray , but the way it is typically ... 145, right_vel, left_vel) ser.write(cmd) ser.flush().
#13. psSerial: how to write a single byte ... - [email protected]
Why do you assume that? Its not said anywhere, and its wrong. A string consists only of the characters you can "see" - if python usues C
#14. pySerial Documentation - Read the Docs
Debian/Ubuntu: “python-serial”, “python3-serial” ... Getter Get the number of bytes in the output buffer. Type int. Platform Posix.
#15. pyserial read write example - gists · GitHub
pyserial read write example. GitHub Gist: instantly share code ... import serial.tools.list_ports as port_list ... serialPort.write(bytes.fromhex("A551F6")).
#16. Sending null byte to serial port in Windows using PySerial?
com.write(chr(0)). The device should reply a single byte upon receiving this byte, but I never got anything in reply. I looked at PySerial's code, ...
#17. [Python教學#1] Windows外接USB轉RS232 Serial並使用 ...
醬是創客的Python教學主題第1篇,以Python語言來實作教學,本篇教學將以Windows 10外接USB轉RS232 Serial並使用python的pySerial套件接收/發送byte實作, ...
#18. Everything You Should Know About Python Serial Read
It provides the number of bytes in the output buffer. Here's an example program implementing the said function. 01. 02. 03. 04.
#19. Sending 4 bytes Hex data over serial in PyQt - Python Forum
I would like to send 4 Bytes of Hex data over serial to a ... Then you can send the bytestring via serial.write(my_bytestring1).
#20. python serial port write byte array - 掘金
python serial port write byte array技术、学习、经验文章掘金开发者社区搜索结果。掘金是一个帮助开发者成长的社区,python serial port write byte array技术文章由 ...
#21. python serial 模組使用方法#1 - 老工程師的練功坊
write (data):傳送data,並返回傳送位元組數。如果bytes和bytearray可用(python 2.6以上),則接受其作為引數;否則接受str作為引數。
#22. Communicating with embedded systems using Python
If you don't know, pySerial (imported as serial ) is a library that ... expects and returns bytes , you'll need to convert Python int s, ...
#23. SerialPort.Write Method (System.IO.Ports) - Microsoft Learn
Writes data to the serial port output buffer. In this article. Definition; Overloads; Write(String); Write(Byte[], Int32, Int32); Write ...
#24. 串行通信- 字節流(Serial Communication - byte stream)
_Comport.Write("B8,4D,18,30,20,B8");. I also attempted to convert the command to a byte array, however ...
#25. Help with pyserial and sending binary data? - Python - Bytes
import serial, string output = " " ser = serial.Serial('/dev/ttyUSB0', 4800, 8, 'N', 1, timeout=1) ser.write(chr(0x80)+chr(0x10)+chr(0xF0)+chr(0x01)+ ...
#26. Cross Platform serial communication using Python (PySerial ...
Here A is defined as a byte by using b prefix.You can also use the bytearray() function. SerialObj.write(b'A') #transmit ...
#27. Python serial write to SAMD21 - serial breaks after one buffer ...
Have you used a debugger to see what's actually happening in the Python? In my opinion printing the bytes that I am sending is good enough - it ...
#28. Serial read with python - Discussions on Python.org
b'\x00' is a single byte (it is a single character in bytes as well as in str ), not two · '\x00' or we can write it as '\0' is ASCII NUL ...
#29. serial.write - Reference / Processing.org
Writes bytes, chars, ints, bytes[], Strings to the serial port ... Serial.list()[0], 9600); // Send a capital "A" out the serial port myPort.write(65); ...
#30. tools/telemetry/third_party/pyserial/serial/serialutil.py
Python Serial Port Extension for Win32, Linux, BSD, Jython ... """convert a sequence to a bytes type""" ... """Write timeouts give an exception""".
#31. Raspberry Pi UART Communication using Python and C
Bytes read from the port. write(Data). This function is used to transmit/send data on Serial Port. Parameter: Data – Data to send.
#32. Data from Teensy 4.0 to Python via serial
Thread: Data from Teensy 4.0 to Python via serial ... byte first Serial.write(b[1]); Serial.write(b[2]); Serial.write(b[3]); } } }.
#33. Arduino Serial.read( ) and Serial.write( ) - Javatpoint
It sends the binary data to the serial port in Arduino. The data through Serial.write is sent as a series of bytes or a single byte. The data type is size_t.
#34. 使用Python的pySerial模組進行序列通訊:連接電腦與Arduino ...
pySerial 提供初始化序列埠、傳送和接收序列數據的指令,像read(), readline()和write()基本指令名稱和語法,跟MicroPython的UART模組一樣。
#35. Python – Pyserial string to byte encoding to write to ... - iTecNote
Python – Pyserial string to byte encoding to write to microcontroller. pyserialpythonserial-communication. I'm trying to communicate between my PC and a ...
#36. Arduino Serial Communication - HKU Robocon Tutorials
No worry, you can write your own Serial Monitor using Python! pySerial is a Library which allows python programs to access the serial port. Thus, we can now ...
#37. python 3.5 中pyserial串口通信如何向串口发送十六进制 ... - 知乎
已解决。 发送十六进制指令先将十六进制数转化为十进制值,然后通过ser.write()发送。在python中,只有bytes类型能通过串口收发,转化的实质是将十六进制代码逐字节 ...
#38. Read from and write to a serial port - web.dev
What is the Web Serial API? #. A serial port is a bidirectional communication interface that allows sending and receiving data byte by byte.
#39. how to send bytes to serial port? - Python - Ubuntu Forums
#!/opt/bin/python import serial, string def write_port(data): #usage ... Serial('/dev/usb/tts/2', 115200, timeout=1) print "write bytes.
#40. Serial Port Communication between PC and Arduino using ...
Do Like and Subscribe Time Stamps BelowSerial Port Programming tutorial using Python and PySerial Library for Embedded developers.
#41. Raspberry Pi Arduino Serial Communication - Everything You ...
Raspberry Pi Arduino Serial communication - with complete Python code example. Learn how to connect your boards together, setup software, and write code.
#42. tbot_contrib.connector.pyserial — tbot 0.10.1-29-gc948daa ...
Serial (os.fspath(port), baudrate=baudrate, exclusive=True) self.serial.timeout = 0 def write(self, buf: bytes) -> int: if self.closed: raise channel.
#43. How to use the SERIAL ports - Acme Systems
Python3 examples. Send a sequence of bytes import serial import time ser = serial.Serial( port='/dev/ttyUSB0', baudrate=9600, timeout=1, parity=serial.
#44. Arduino Serial Read Write Structure With Code Examples
The first byte of incoming serial data available (or -1 if no data is available). Data type: int . What is the difference between serial write and serial print ...
#45. Python Examples of serial.to_bytes - ProgramCreek.com
This page shows Python examples of serial.to_bytes. ... Check bytes written bytes_written = ser.write(serial.to_bytes(serial_data)) # Wait before checking ...
#46. psSerial: how to write a single byte value to the serial port?
http://pyserial.sourceforge.net/ example shows how to write string to serial port. #Open port 0 at "9600,8,N,1", no timeout how do i write a ...
#47. Python Datalogger - Using pySerial to Read Serial Data ...
Python Datalogger - Using pySerial to Read Serial Data Output from ... are reading all the bytes that are being outputted by the Arduino.
#48. 使用python下的pyserial进行串口测试和设备调用 - MCU加油站
sudo pip3 install pyserial. 2、Bytes与string的转换. 很多串口使用的数据是byte格式,需要进行转换。 使用str.decode()将ASCII转为String的Unicode ...
#49. Triggering from Serial port - Coding - PsychoPy Discourse
General purpose communication with serial devices using Python is usually ... Something like this will write a byte to the port and read the ...
#50. How do I get VB.Net to use WriteByte() - CodeProject
The Serial Port go-to book Serial Port Complete 2nd Ed by Jan Axelson says Yes, but the IDE reports WriteByte is not a member of 'System.IO.
#51. Tips for reading a serial data stream in Python - BEC Systems
The easiest way to get python talking to serial ports is use the pyserial ... will be best if we can read more than one byte at a time.
#52. Serial UART communication on RPi's TTL port
Learn to use PySerial library for serial UART between Raspberry Pi and a ... serial.write() — This method writes byte data to the serial port (and the data ...
#53. PySerial - How to send numbers larger than 256?
However there is a serial receive buffer which holds up to 64 bytes, ... s.pack(x) # Write the raw, binary float to the serial port. ser.write(packedData) ...
#54. Linux Serial Ports Using C/C++ - mbedded.ninja
c_oflag &= ~OPOST; // Prevent special interpretation of output bytes (e.g. newline chars) tty.c_oflag &= ~ONLCR; // Prevent conversion of ...
#55. Frames and protocols for the serial port - in Python
I specifically decided to write them as separate posts and not as part ... When we think about a sequence of bytes in Python, two approaches ...
#56. Using Python and an Arduino to Read a Sensor
Write a Python script to print out the potentiometer readings; Write a ... To communicate with the Arduino using Python over a serial line, ...
#57. Python 3 Unicode and Byte Strings - Sticky Bits - Feabhas
It is quite likely that when migrating existing code and writing new ... pySerial you will find that data is now stored as byte strings.
#58. Reading Arduino serial ports in Windows 7 with Python + ...
Hello world with serial ports. Arduino code: · Arduino read data from Python. Arduino read data when user type something and prints it out byte ...
#59. SER_README.txt
pySerial -------- This module capsulates the access for the serial port. ... blocking or non-blocking - file like API with "read" and "write" ("readline" ...
#60. How to Convert Bytes to Int in Python? - GeeksforGeeks
A bytes object can be converted to an integer value easily using Python. Python provides us various in-built methds like from_bytes() as ...
#61. Unable to send byte from Python serial.write() to Arduino
Per the Pyserial documentation: write(data) Parameters: data – Data to send. Returns: Number of bytes written. Return type: int Raises: ...
#62. send integer to serial port - General - Xojo Programming Forum
The Serial.write function takes only characters. Do I convert to 2 bytes first then send each byte as a character? if so how do I converts?
#63. Using Python's asyncio with serial devices - Tinkering
Once you have the Serial object you can send or receive bytes from the serial port via the Serial.write and Serial.read methods respectively ...
#64. IWR1443BOOST: Reading via Serial with Python - TI E2E
IWR1443BOOST: Reading via Serial with Python ... 8, 7] magicWord = [bytes(i) for i in magicWord] # Byte sizes for different values magicWord ...
#65. class UART – duplex serial communication bus
Write the buffer of bytes to the bus. Return value: number of bytes written or None on timeout. UART.sendbreak ...
#66. PyserialでのBinary送受信 - Qiita
この中で、pyserialのSerial.write()メソッドは文字列データのみを送信する。 ... PythonのBinaryに変換 bytesを用いた方法b2_data = bytes(b) ...
#67. Interpreting Serial Data – ITP Physical Computing
Serial data is passed byte by byte from one device to another, but it's up to you to ... In Arduino, you can do this using the Serial.write() command, ...
#68. Solved: Sometimes bytes lost, when reading from serial port
Solved: Hello! I'm reading data from serial port (baud rate 57600) which works very reliable as long as I do not open any other window or.
#69. Serial Communication between Raspberry Pi & Arduino
Python Code Now we need to ask Raspberry Pi to write data to Arduino We will use ser.write function this time. It simply writes one byte of ...
#70. Using PySerial, PyNMEA2, and Raspberry Pi to log NMEA ...
Using PySerial, PyNMEA2, and Raspberry Pi to log NMEA output ... is a way to make sure that the bytes objects are defensively handled.
#71. Develop a serial monitor with Python - AranaCorp
The Arduino code for serial communication is quite simple, ... () >0) { char c = Serial.read(); //gets one byte from serial buffer msg += c; ...
#72. Serial port programming - eLinux.org
We will now write a simple Python program which we can talk to with the terminal emulator. You will need to install the PySerial package:
#73. Serial Communication: Sending a 10-Bit (or two-byte) Value
Serial Communication : Sending a 10-Bit (or two-byte) Value. When communicating between a microcontroller and a personal computer using ...
#74. Can you please help me to understand these questions?
32 lines (28 sloc) 714 Bytes Raw Blame CP 1 /* Serial Transmit Example 2a: send data type byte, unsigned 8 bit * with write command. 2 3 4 Serial.write ...
#75. Send Unsigned Byte Over Serial - Java - Chief Delphi
Testing with python I could send a byte equal to 255 which would be received as 255 by the arduino, so I'm unaware of a way to do it in Java. So far the ...
#76. How do I dump every byte written to a tty? - Super User
If I connect using the Python pyserial library and write a few line breaks ... I can then read and write bytes using pyserial perfectly.
#77. Useful Python Script to Send and Receive Serial Data
In my case, my serial port was serial0. We can now write a simple script to write data every 1 second: import time import serial ser = serial.
#78. Send a byte from a C program to the serial port to be read by ...
Hi Im trying to write a C program using Mc18 to send a byte to the ... 4)to access the serial port you will need PySerial Module (link to ...
#79. NION Python NULL Byte for Serial Port? (Page 1)
I can send each byte individually, one line of Python per byte (port.write("\xF5") etc), but then only the non-null bytes are sent out the ...
#80. 파이썬(Python) pyserial을 이용한 UART 바이트(Byte) 데이터 ...
import serial # pyserial 라이브러리 가져오기 def sendData(x,y): # 1 Byte 보내기 16진수 0xC0 보내기 ser.write(bytes(bytearray([0xC0]))) # 1 ...
#81. ESP32 / ESP8266 Arduino: Serial communication with Python
ESP32 / ESP8266 Arduino: Serial communication with Python ... Thus, we can write a loop that will keep reading the bytes of the Serial port ...
#82. [Example code]-Send Byte serially using PySerial in Python 3
The write() function accepts only sequences (bytesarray, bytes, etc..). If I cast my binary value to bytesarray(), it's going to return an array of values (0- ...
#83. Introduction to Python Serial Ports | PIC - Maker Pro
read(size) – This will read n number of bytes from the serial port; write(data) – This will write the data passed to the function to the ...
#84. Writing bytes to a serial port in C
I have a serial device that looks for a simple 4-character command, e.g. 'abcd' to change the kind of data it sends. With the python client ...
#85. 安裝pySerial - 芭蕉葉上聽雨聲
您可以使用pySerial 透過COM port 與Arduino 聯繫。 ... s = ser.read(10) # read up to ten bytes (timeout) ... sp.write(chr(1))
#86. Write data to serial port - MATLAB write - MathWorks
Write and Read Data with Serial Port Device ... the number of bytes to write for each value and the interpretation of those bytes as a MATLAB data type.
#87. Bytes to hex python - Istabagno
Write a Python program to convert an integer to a 2 byte Hex value. ... there is no bytes are sended, only ascii codes #!/opt/bin/python import serial, ...
#88. Serial Communication Using Python - Embedded Laboratory
Python doesn't have any library for serial communication, so before proceeding ... ser.write(temp) #Writes to the SerialPort while 1: bytes ...
#89. serial port read timeout linux - Le Camere di Corte
ReadTimeoutError: HTTPSConnectionPool(host='files. i read this question Read 0 bytes after writing to /dev/ttyS0 i want a sample like this in cpp. 1. 2019.
#90. ANSI escape code - Wikipedia
Certain sequences of bytes, most starting with an ASCII escape character and a bracket character, are embedded into text. The terminal interprets these ...
#91. python通過pyserial讀寫串列埠 - 程式人生
port # port name/number as set by the user; baudrate # current baud rate setting; bytesize # byte size in bits; parity # parity setting ...
#92. Serial To Hex - Rücken und Energie Anita Grossenbacher
For all the text characters you should get the hex bytes: "50 6C 61 6E 74 20 74 72 65 ... Resolved [RESOLVED] Serial Communication / HEX to ASCII conversion ...
#93. how can i send a byte array to a serial port using python?
Changed in version 2.5: Accepts instances of bytes and bytearray when ... with serial.serial_for_url(port) as s: ...s.write(b 'hello').
#94. Challenges - Coderbyte | The #1 Coding Assessment Platform
Time Convert. Not Completed. Easy. Solutions: 94290. Alphabet Soup. Not Completed ... Solutions: 4123. Serial Number. Not Completed. Easy. Solutions: 6381.
#95. Getting Started with Python and Raspberry Pi
Now that the port is created, we can send some data as a Python string. port.write('Hello, world!') Next, we will read a byte from the serial port at a time ...
#96. python read binary file - Orizzonti consulting
Create a python file with the following script. Write a program to read data from file “data. Read bytes from Binary File Now you can easily read the file ...
#97. I2c read
WriteByte (Byte) Writes a byte to the I2C device. ... In this tutorial, we will use the oscilloscope to read an I²C serial bus. 1 i2c_read.
python serial write byte 在 Serial Port Communication between PC and Arduino using ... 的美食出口停車場
Do Like and Subscribe Time Stamps BelowSerial Port Programming tutorial using Python and PySerial Library for Embedded developers. ... <看更多>