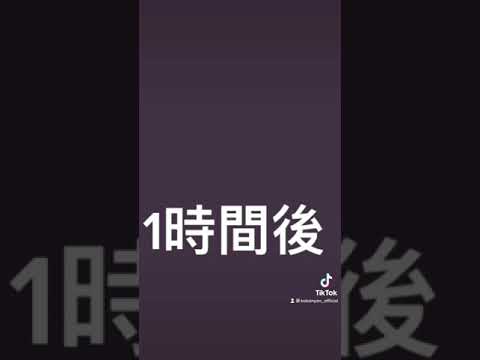
find median algorithm 在 コバにゃんチャンネル Youtube 的最讚貼文
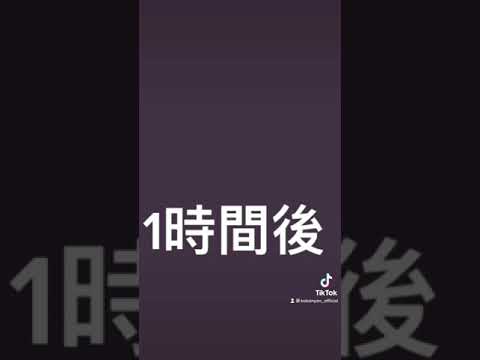
Search
#1. My Favorite Algorithm: Linear Time Median Finding
The most straightforward way to find the median is to sort the list and just pick the median by its index. The fastest comparison-based sort is ...
Compare all n-1 elements with the median of medians m and determine the sets L and R, where L contains all elements <m, and R contains all elements >m.
#3. Median of an unsorted array using Quick Select Algorithm
Given an unsorted array arr[] of length N, the task is to find the median of this array. Median of a sorted array of size N is defined as ...
#4. Find median of unsorted array in $O(n)$ time
The main idea of the algorithm is to use sampling. We have to find two elements that are close together in the sorted order of the array and ...
#5. Median of medians Algorithm - [Linear Time Complexity O(n ...
Median of medians can be used as a pivot strategy in quicksort, ... as you just need to find the median, there's no need to know the sorted ...
#6. Median Finding Algorithm - by Jemutai Sitienei - Medium
Algorithm. Using divide and conquer strategy, first let us find an element x and break S into two arrays B and T such that every element in B is ...
#7. Median of medians - Wikipedia
In computer science, the median of medians is an approximate median selection algorithm, frequently used to supply a good pivot for an exact selection ...
#8. Finding the Median in Linear Time
We would like to compute their median in linear time. This can be done in linear time by a relatively complicated determinsitic algorithm (see any standard ...
#9. 4.3. Median Sort - Algorithms in a Nutshell [Book] - O'Reilly
Median Sort Divide and conquer, a common approach in computer science, solves a problem by dividing it into two independent subproblems, each about half the ...
#10. Median of Medians Algorithm - OpenGenus IQ
This algorithm runs in O(n) linear time complexity, we traverse the list once to find medians in sublists and another time to find the true median to be used as ...
#11. Algorithms Chapter 9 Medians and Order Statistics
How can we find the ith order statistic of a set and what is the running time? ... The algorithm is optimal, because each element, except the.
#12. Median of Array (Unsorted) - Scaler Topics
Algorithm · Create a function to find the median of an array that takes the array. · First, we will sort the given array. · Then check for even ...
#13. What is an algorithm for finding the median number of three ...
NOW FIND THE MEDIAN (this is the sum of the three numbers, less the minimum and maximum):. median := a+b+c - minimum - maximum.
#14. Finding the median of an unsorted array - Stack Overflow
max()+MinHeap.min())/2 this takes O(1) also. Thus, the real cost of the whole operation is the heaps building operation which is O(n).
#15. Find Median from Data Stream - LeetCode
Can you solve this real interview question? Find Median from Data Stream - The median is the middle value in an ordered integer list. If the size of the ...
#16. Deterministic selection
Median of medians · Line up elements in groups of five (this number 5 is not important, it could be e.g. 7 without changing the algorithm much). · Find the median ...
#17. C program to find the median of a given list - Tutorialspoint
Refer an algorithm given below to calculate the median. Step 1 − Read the items into an array while keeping a count of the items. Step 2 − ...
#18. Find median of an array using Quick Select Algorithm in C++
1. First, pick a pivot element randomly from arr[] then use the partition step from the quick sort algorithm. · 2. Then after the above step, it is the median of ...
#19. Median value of array - MATLAB median - MathWorks
Find the median values of this 3-D array along the second dimension. M = median(A). M = M(:,:,1) = 9 M ...
#20. Selection (deterministic & randomized): finding the median in ...
randomized): finding the median in linear time. 4.1 Overview. Given an unsorted array, how quickly can one find the median element?
#21. An Efficient Algorithm for the Approximate Median ... - DMI Unict
in-place median finding algorithms have been proposed. ... cient to find an approximate median, for example in some heapsort variants (cf. [Ros97],.
#22. The Selection - Introduction to Algorithm Analysis
A Lower Bound for Finding the Median ... to find an element with the kth smallest key in E. ... fewer than n-1 comparisons to find the largest entry.
#23. Find minima and maxima using the median of ... - Swift Forums
Currently the minima and maxima functions use @soroush's algorithm, which has expected running time of O(k log k + nk), where n is the ...
#24. Find Median of 1D Array Using Functions in C - Javatpoint
Algorithm · First, read the items into an array while maintaining an item count. · The objects are sorted in step two by increasing value. · Calculate the median.
#25. Median-of-Medians Algorithm: Pros and Cons for Quicksort
The median-of-medians algorithm is a recursive method to find the median of a set of numbers in linear time. The median is the middle element of a sorted ...
#26. Median of Two Sorted Arrays - InterviewBit
Find the median of the two sorted arrays( The median of the array formed by ... The merging of two sorted arrays is similar to the algorithm ...
#27. Is there an algorithm to find the median of a very large stream ...
An algorithm that only does one pass on data is usually called a streaming algorithm: ... If I have to find the median of a list of 1 million numbers, ...
#28. Averages/Median - Rosetta Code
Task Write a program to find the median value of a vector of ... The best solution is to use the selection algorithm to find the median in O(n) time.
#29. Suppose we have a O(n) time algorithm that finds ... - EduRev
Now consider a QuickSort implementation where we first find median using the above algorithm, then use median as pivot. What will be the worst case time ...
#30. Solved In the median finding algorithm, suppose in step 1
we divide the input into blocks of size 7 each and find the median of the median of blocks and proceed, does that result in a linear algorithm. In the median ...
#31. median-of-medians.md - GitHub Gist
Median of medians is an algorithm to select an approximate median as a pivot for a partitioning ... :param arr: Array from which we need to find the median.
#32. Quicksort with median of medians algorithm(efficient sorting)
The median-of-medians algorithm is a deterministic linear-time selection algorithm. Using this algorithm, we can improve quick sort algorithm!
#33. How To Find the Median of a Data Set in Statistics | Indeed.com
Finding the median of an even number of data points requires an extra step: Order all numbers from lowest to highest. Count how many numbers are ...
#34. An improved algorithm for finding the median distributively
Given two processes, each having a total-ordered set ofn elements, we present a distributed algorithm for finding median of these 2n elements using no more.
#35. algorithm to calculate the median for a sorted array - delphi
algorithm to calculate the median for a sorted array. Hi,. I'm new at algorithms and i'm trying to do an algorithm to calculate the median ...
#36. How to Find the Median | Definition, Examples & Calculator
The steps for finding the median differ depending on whether you have an odd or an even number of data points. If there are two numbers in the ...
#37. The Median-of-Medians Algorithm - Austin Rochford
The Median-of-Medians Algorithm · Select a pivot element, and partition the list into two sublists, the first of which contains all elements ...
#38. Running Median Algorithm and Implementation for Integer ...
Abstract—A novel algorithm is proposed to compute the median ... heaps [5] and skip lists [6] with O(lg m) time at best to find the median per iteration.
#39. Python statistics.median() Method - W3Schools
Calculate the median (middle value) of the given data: # Import statistics Library import statistics # Calculate middle values print(statistics.median([1, ...
#40. Median queries | Practice Problems - HackerEarth
Advanced Algorithms, Algorithms, Approved, Easy, Grammar-Verified, Recruit, ... You are given two integers L and R. You have to find the median of a ...
#41. Program for Mean and Median of an unsorted array
We can calculate the mean and median by the formula given above ... The space complexity of an algorithm is the total space taken by the ...
#42. CS161: Algorithm Design and Analysis Recitation Section 3
median -of-3 method, the pivot is chosen as the median of a set of 3 elements ... Solution: Use the deterministic selection algorithm to find the median.
#43. CSE101: Algorithm Design and Analysis - UCSD CSE
Median (algorithm). • Can you think of an efficient way to find the median? • How long would it take? • Is there a lower bound on the runtime of all median.
#44. Algorithm to find median from stream of correlated numbers?
Hi all, This isn't really a rust-specific question, but this is my best place to look for help. :slight_smile: I'm wanting to estimate the ...
#45. What's the fastest algorithm to solve this problem? - Codeforces
Find the nearest 1 million stars from the earth. ... Then the median of medians algorithm finds the k-smallest element in O(n) time worst-case, ...
#46. 4.4.2 Calculating the median - Statistique Canada
The median is the value in the middle of a data set, meaning that 50% of data points have a ... How would you calculate his median time?
#47. Find median of two sorted arrays - IDeserve
Here is a video that explains algorithm in log(n) time for finding median of two sorted arrays with the help of examples and animations.
#48. Calculate the Median of an Array in JavaScript - Mastering JS
Here's how you can quickly calculate the median of an array of numbers in vanilla JavaScript, with no outside libraries.
#49. An algorithm for solving the bi-objective median path-shaped ...
The algorithm is based on the two-phase method which can compute all Pareto solutions for the BMP problem. The first phase is applied to find ...
#50. Java Program To Calculate Median Array | 4 Methods
Java code To Calculate Median – In this article, we will brief in on the mentioned means to Finding the middle element in array.
#51. Binapprox median algorithm · 2020 - Coda
Binapprox algorithm exploits this fact to find the approx median. . It is a fast algorithm to find the approximate running median of a data set.
#52. Median: How to Find it, Definition & Examples - Statistics How To
A caution with using the median formula: The steps differ slightly depending on whether you have an even or odd amount of numbers in your data set. Find the ...
#53. A Linear Time Algorithm for the Weighted Median Problem ...
tational experience with the linear median algorithms for both the unweighted ... find the kth smallest element of the n elements in the set S.
#54. Python: Compute the median of all elements - w3resource
Python heap queue algorithm: Exercise-16 with Solution. Write a Python program that adds integer numbers from the data stream to a heapq and ...
#55. A Self-Stabilizing Distributed Algorithm to Find the Median of a ...
We propose a self-stabilizing algorithm (protocol) for computing the median in a given tree graph. We show the correctness of the proposed algorithm by ...
#56. An optimal quantum algorithm to approximate the mean and ...
We then propose a new algorithm for approximating the median of a set of points ... algorithm that can find the minimum of a function with a ...
#57. Median of Medians Algorithm | Interview
1. Divide the list into sublists of length five. · 2. Sort each sublist and determine its median directly. · 3. Use the median of medians algorithm to recursively ...
#58. Running Median - Data Structures and Algorithm
Find the running median after entering every number from a sequence of infinite integers. Brute force. If we keep each number in a sorted ...
#59. On the median-of-k version of Hoare's selection algorithm
Let C^ j be the (random) number of comparisons required by FIND({1, ... , n},l) if, in each recursion step, the partitioning element is the médian of 2/c + 1 ...
#60. Quick Sort - Algorithms
Suppose we have a O(nlogn) time algorithm that finds median of an unsorted array. Now consider a QuickSort implementation where we first find ...
#61. suppose we have a0(n) time algorithm that finds median of an ...
Now consider a QuickSort implementation where we first find median using the above algorithm, then use median as pivot.
#62. Improved Greedy Algorithm for Computing Approximate ...
To find a median string is an NP-Hard problem in general, so it is useful to develop fast heuristic algorithms that give a good approximation of the median ...
#63. Median-Median Line Algorithm on TI Graphing Calculators.
What method is used to calculate the median-median line? · 1) Divide the data into three parts with an equal number of data points · 2) Define a summary point for ...
#64. Use the Find Largest algorithm to help you develop an algori
The median of N numbers is defined as the value in the list in which approximately half the values are larger than it and half the values are smaller than it.
#65. numpy.median — NumPy v1.24 Manual
Axis or axes along which the medians are computed. The default is to compute the median along a flattened version of the array. A sequence of axes is ...
#66. hw3 sol.pdf - CS6515 – HW3 Problem 1 Median algorithm Let...
Solution:I would run the divide and conquer median find algorithm.It returns the kth number in the array ((3n/5)<k<(7n/5)) and if(k) lies in (n-k)/2 to ...
#67. find median from data stream - median in a stream of integers
Algorithm · if the current element is greater than the median value, insert it into min heap.return the root of minheap as the new median. · else if the current ...
#68. Fast median algorithm (Read 32864 times)
mov edx,eax ; Save the first middle number to calculate the average of 2 middle numbers. FindSecondMiddleNumberLP: inc eax movzx ecx,byte ptr[ ...
#69. Median Implementation in C# - Programming Algorithms
Median Programming Algorithm in C#. This algorithm computes the median of the given set of numbers.
#70. How to Find Median for Grouped and Ungrouped Data - Byju's
Then find the class whose cumulative frequency is greater than and nearest to n/2. This is the median class. Step 6. Now we use the formula.
#71. PCM Omars Algorithm: Parallel computation of Median
[email protected]. Abstract: the goal of a distributed computation algorithm is to determine the result of a function of numerical elements, which ...
#72. MEDIAN function - Microsoft Support
This article describes the formula syntax and usage of the MEDIAN function in Microsoft Excel. Description. Returns the median of the given numbers.
#73. An Efficient Algorithm for the Approximate ... - Computer Science
Most of the work of the algorithm is spent in TRIPLET ADJUST, comparing values and exchanging ele- ments within triplets to locate their medians ...
#74. A Modified Median String Algorithm for Gene Regulatory Motif ...
Consensus string is a significant feature of a deoxyribonucleic acid (DNA) sequence. The median string is one of the most popular exact algorithms to find ...
#75. Calculating Mean, Median, and Mode in Python - Stack Abuse
Let's take a look at how we can use Python to calculate the median. Finding the Median With Python. To find the median, we first need to sort ...
#76. Formula to Calculate Median in Statistics - WallStreetMojo
Median Calculation (Step by Step) · Firstly, sort the numbers in ascending order. · The method of finding a median of the odd/even numbers in the group is below.
#77. A Simple Linear Time Algorithm for Computing a 1-Median on ...
Therefore, from here on we only focus on vertex of the graph in order to find a 1-median. We recall the definition of trees and cactus graphs. A tree is an ...
#78. A Monte Carlo algorithm to estimate a median - The DO Loop
For example, if you want to find the median value of 10 billion credit card transactions, it might not matter whether the median $16.74 or ...
#79. A fast algorithm for two dimensional median filtering
0098-4094,'87/1100-1364$01.00 01987 IEEE. Page 2. AHMAD AND SUNDARARAJAN: ALGORITHM FOR 2-D MEDIAN FILTERING. 1365 procedure to find median results in faster ...
#80. An Improved Approximation Algorithm for Knapsack Median ...
We also give a polynomial-time algorithm to sparsify any knapsack median ... is an efficient algorithm based on the ellipsoid method to find such a de-.
#81. How to Use median() in NumPy? - Spark By {Examples}
median () function is used to calculate the median of single-dimensional as well as multi-dimensional arrays. In this article, I will explain how ...
#82. Apply the partition-based algorithm to find the median of the ...
VIDEO ANSWER: We recall that the definition of median is the middle number and a set of data when we are asked to find it.
#83. [Solved] Lets say that we have an On time algorithm ... - Studocu
On Studocu you find all the lecture notes, summaries and study guides you need ... Let's say that we have an O(n) time algorithm which looks for the median ...
#84. Median of Grouped Data - Formula, Steps and Examples
The median is the middle value of a set of data. To find the median of a data group, arrange the data in order of magnitude and then find ...
#85. quantile algorithm
3. Unbiased quantiles. To get a better estimate of the quantiles of the underlying distribution, the interpolation that we used to determine the median, is ...
#86. A Fast Two-Dimensional Median Filtering Algorithm - UiO
The algorithm consists of the following steps: Step I: Set up the gray level histogram of the first window and find the median. Also, make the.
#87. Here are several problems that are easy to solve in O(n) time ...
Describe a fast algorithm to find the median (meaning the nth smallest element) of the union A∪ B. For example, given the input. A[1 .. 8]=[0, 1, 6, 9, 12, ...
#88. Finding Missing Data Values given the Median and the Range ...
Two values are missing from the shown data set. Given that the median is 67, the range is 44, and the data is listed in ascending order, find ...
#89. Does somebody know efficient algorithm for moving median?
Algorithm : From the sorted array, remove the value with age N; For the new value to be added, use a binary search to find its ...
#90. [PDF] Analysis of Hoare's FIND algorithm with Median-of-three ...
The Median-of-three version of Hoare's Find, where the pivot element is chosen as the median of a random sample of three elements is ...
#91. Online Algorithm - mean and median | yokolet's notelets
This post discusses so-called Online Algorithm. If fixed number of integers (or real numbers) are given, it's easy to find a mean or median.
#92. Median Maintenance algorithm implementation using ...
The median maintenance problem is a common programming challenge presented in software engineering ... Find the repeated item in an array using TypeScript.
#93. Puzzle #??: Optimal median-finding and sorting algorithms
To find the median item? (If N is even, then for our purposes here "median" shall mean "the lesser of the two medians.") Solve this for N=1,2,3,...,13. Assume ...
#94. An Efficient Fuzzy C-Least Median Clustering Algorithm
An Efficient Fuzzy C-Least Median Clustering Algorithm. Moksud Alam Mallik 1, Nurul Fariza Zulkurnain 2, Mohammed Khaja Nizamuddin 3 and Aboosalih KC 4.
#95. Median of medians - Programming
The median-of-medians algorithm does not actually compute the ... Find the median of the x[i], using a recursive call to the algorithm.
#96. CS584/684 Algorithm Analysis and Design – Spring 2017 ...
Invoke MOM-SELECT recursively on the ⌈n/5⌉ medians to obtain pivot value x (the “median of medians”). 4. Determine the index i of x in the ...
#97. A fast weighted median algorithm based on Quickselect
Further denote W[k] as the associated weight of X(k). Quickselect is an algorithm to find the kthOS of a set of samples. Page 6. Outline.
find median algorithm 在 Median of medians Algorithm - [Linear Time Complexity O(n ... 的美食出口停車場
Median of medians can be used as a pivot strategy in quicksort, ... as you just need to find the median, there's no need to know the sorted ... ... <看更多>