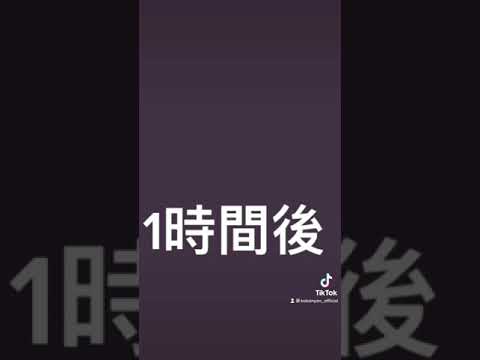
assign string to char 在 コバにゃんチャンネル Youtube 的精選貼文
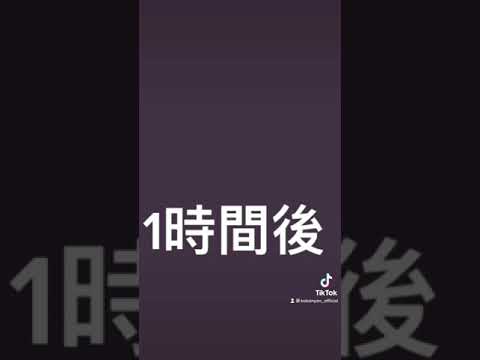
Search
#1. Assigning strings to arrays of characters - Stack Overflow
When initializing an array, C allows you to fill it with values. So char s[100] = "abcd";. is basically the same as int s[3] = { 1, 2, 3 };.
#2. Convert string to char array in C++ - GeeksforGeeks
A way to do this is to copy the contents of the string to char array. This can be done with the help of c_str() and strcpy() function of library ...
#3. string::assign - C++ Reference
string& assign (const char* s, size_t n); ... string& assign (size_t n, char c); ... Assigns a new value to the string, replacing its current contents.
#4. How to "re-assign" a character string to an existing char array?
char myArray[50] = "Testing123"; but I'm asking how to do the assignment after the array is created... or how to clear & re-assign a string ...
#5. assigning string to char array in c++ Code Example
std::string myWord = "myWord"; char myArray[myWord.size()+1];//as 1 char space for null is also required strcpy(myArray, myWord.c_str());
#6. [C/C++] String <-> Char - 黑皮扣丁- 痞客邦
string a;; a.c_str();. 2. Char to String. 方法一: 只要將char當成建構子傳進去即可. ex. char *a="123";; string A(a);. 方法二: 呼叫assign.
#7. String Copy - How to play with strings in C - CodinGame
strcpy can be used to copy one string to another. Remember that C strings are character arrays. You must pass character array, or pointer to character array ...
#8. C++ String to Char Array - Tutorial Kart
Method 1: Assign String Literal to the Char Array · Method 2: Assign Each Character of String to Char Array · Method 3: Use strcpy() and c_str() · Conclusion.
#9. How to Store Strings in C Programming - dummies
Program to Destroy the World Press Enter to explode: A string variable in C is really a character array. You can assign a value to a char array when it's ...
#10. String and Character Arrays in C Language | Studytonight
The gets() function can also be used to read character string with white spaces. char str[20]; printf(" ...
#11. String Assignment
Unlike assignment of char * variables, strings are copied by value, not by reference. String assignment is performed using the = operator and copies the ...
#12. Convert string to char array in C#
string sentence = "Mahesh Chand"; · char[] charArr = sentence.ToCharArray(); · foreach (char ch in charArr) · { · Console.WriteLine(ch); · }.
#13. 4 Ways to Convert String to Character Array in JavaScript
How to convert a string into an array of characters in JavaScript? ... 2 [...string]; // Option 3 Array.from(string); // Option 4 Object.assign([], string); ...
#14. [Solved] C assign string from argv[] to char array - Code Redirect
You must copy the string into the char array, this cannot be done with a simple assignment. The simplistic answer is strcpy(filename, argv[1]); . There is a big ...
#15. C++ Assign String To Char: Detailed Login Instructions
Video result for C++ Assign String To Char · Learn C Programming - Strings (char arrays) Part 1 · PASS STRING/CHAR ARRAY/CHAR POINTER TO FUNCTION IN C |... · How ...
#16. Why assigning string to const char* is possible? - Reddit
Why assigning string to const char* at the initialization is possible (but it's not for normal char*, what is understable since it is a ...
#17. C# Strings - TutorialsTeacher
A variable of the string type can be declared and assign string literal, ... So, string can be created using a char array or accessed like a char array.
#18. Convert a std::string to char* in C++ - Techie Delight
This post will discuss how to convert a `std::string` to `char*` in C++. The returned array should contain the same sequence of characters as present in the ...
#19. Character array - MATLAB - MathWorks
For instance, if A is a string, "foo" , c is a character array, 'foo' . example. C = char(A1,...,An) converts the arrays A1 ...
#20. Why does assigning a string literal to a char pointer prevent ...
Why does assigning a string literal to a char pointer prevent you from being able to modify individual characters of the string, in C? 4 Answers.
#21. The Basics of C Programming - Strings - Computer ...
Pascal can simply return the length byte, whereas C has to count the characters ... For example, suppose you want to assign one string to another string; ...
#22. Strings in C: How to Declare Variable, Initialize, Print, Example
char first_name[15]; //declaration of a string variable char last_name[15];. The above example represents string variables with an array ...
#23. 【C++】字串char string stringstream 相關用法總整理(內含範例 ...
(與利用sprinf, snprinf,assign值的方法), 其中char 我們又會分成char array, char pointer 介紹,以及會介紹他們 ...
#24. Char array to struct c - Reald2
Therefore, you need an 8 character array to told a 7 character string. Next, I am assigning value in structure members with the help of for loop and ...
#25. C++ std::string::assign()用法及代碼示例- 純淨天空
string & string::assign (const char* cstr) Assigns all characters of cstr up to but not including '\0'. 返回: *this. Note: that cstr may not be a null pointer ...
#26. how to assign string to char array in yacc code example
CPP program to convert string // to char array #include <bits/stdc++.h> using namespace std; // driver code int main() { // assigning value to string s ...
#27. Error: Assign string to the char variable in C - Includehelp.com
Error: Assign string to the char variable in C | Common C program Errors. Here, we will learn how and what an error or warning occurs, ...
#28. How to convert string to char array in C++? - Tutorialspoint
Begin Assign a string value to a char array variable m. Define and string variable str For i = 0 to sizeof(m) Copy character by character ...
#29. Strings and Characters — The Swift Programming Language ...
Swift's String and Character types provide a fast, Unicode-compliant way to work ... a longer string, either assign an empty string literal to a variable, ...
#30. C-String Assignment - NCSU COE People
char example[100] = "First value";. However, it is not legal to assign string variables, because you cannot assign to an entire array. myString ...
#31. Is initializing a char[] with a string literal bad practice?
but it's easier on the eyes to use string literals. EDIT 2. In order to assign the contents of an array outside of a declaration, you would need to use either ...
#32. C++ Tutorial: String - 2020 - BogoToBogo
"Hello" is a string literal, and we may want to use the following to assign it to a pointer: const char *pHello = "hello"; · To store C++ string to C-style ...
#33. std::basic_string<CharT,Traits,Allocator>::assign
This range can contain null characters. 6) Replaces the contents with those of null-terminated character string pointed to by s . The length of ...
#34. string - Arduino Reference
you can use the String data type, which is part of the core as of version 0019, or you can make a string out of an array of type char and null- ...
#35. String to char array java - convert string to char - JournalDev
First two parameters define the start and end index of the string; the last character to be copied is at index srcEnd-1. The characters are copied into the char ...
#36. 如何在C++ 中把Char 陣列轉換為字串 - Delft Stack
使用 std::string 建構函式將char 陣列轉換為string; 使用 memmove 函式將Char 陣列轉換為字串; 使用 std::basic_string::assign 方法將Char 陣列轉換 ...
#37. assign a string to char pointer - C Board
how would i assign a char pointer the value returned by a function that returns a string? Code: [View]. #include <stdio.h> const char *str ...
#38. why we can't assign a string value to 2d char array? - py4u
... char a[3][5]; int i; a[0][5]="hai"; a[1][5]="cool"; a[2][5]="many"; for(i=0;i<3;i++) printf("%s ",a[i]); return 0; }. Why we cant assign string value ...
#39. Java Convert String to char - javatpoint
Java String to char Example: charAt() method · public class StringToCharExample2{ · public static void main(String args[]){ · String s="hello"; · for(int i=0; i<s.
#40. How to convert a char array to a string in Java?
There are two ways to convert a char array (char[]) to String in Java: 1) Creating String object by passing array name to the constructor 2) Using.
#41. C/C++ - String 用法與心得完全攻略
string 是一個保存char 的序列容器,把字串的記憶體管理責任交 ... 對字串操作非常方便,因此assign()、append()、compare() 等函數,除非一些特殊 ...
#42. Assigning String to Char pointer error - CodeProject
const char *test3="Hello everyone .Please help me my string assigning part to char pointer in a code is not working I have been stucked on ...
#43. SystemVerilog Strings - ChipVerify
Learn how to use SystemVerilog strings with simple easy to understand code ... str.len()); // Assign to tmp variable and put char "d" at index 3 tmp = str ...
#44. C++ Tutorial: 3.2, Strings of Characters.
In C++ there is no specific elemental variable type to store strings of ... it would be valid to assign a string of characters to an array of char using a ...
#45. Assign string to char pointer-understanding char pointers
From http://programmers.stackexchange.com/questions/249554/assigning-strings-to-pointer-in-c This is just the way string literals work in C.
#46. STR30-C. Do not attempt to modify string literals - Confluence
Avoid assigning a string literal to a pointer to non- const or casting a ... #include <stdio.h> #include <string.h> const char *get_dirname(const char ...
#47. String in std
You can append a char to a String with the push method, and append a &str with the push_str method: ... Performs copy-assignment from source .
#48. Assigning string constant to char* without malloc - C / C++
char *str; str="hello"; puts(str); return 0; } Before assigning the string constant "hello" to str, str in this case doesn't points to a valid memory ...
#49. Strings - Manual - PHP
A string is series of characters, where a character is the same as a byte. ... As of PHP 7.1.0, assigning an empty string throws a fatal error.
#50. Character Array and Character Pointer in C - C Programming
The type of both the variables is a pointer to char or (char*) , so you can ... This means string assignment is not valid for strings defined as arrays.
#51. C, assigning a char to a string array. [Archive] - Ubuntu Forums
I think I understand it, but I would be grateful for someone to double check. If I run the following code char* strings; *strings = 'a'; ...
#52. Converting String to array of char and vice versa
Strings in Java: String A; A = "abH"; // Assign string to string variable. How a String is stored in memory: · Now compare with how an array of characters is ...
#53. C strings and C++ strings - PrismNet
char s1[20]; // Character array - can hold a C string, but is not yet a valid C ... The C++ compiler interprets these assignment statements as attempts to ...
#54. C Programming Strings - Programiz
char c[5] = "abcde";. Here, we are trying to assign 6 characters (the last character is '\0' ) to a char array having ...
#55. Char Array In Java | Introduction To Character ... - Edureka
We use the following code to assign values to our char array: ... The following code converts a string into a char array.
#56. TString Class Reference - ROOT - CERN
The underlying string is stored as a char* that can be accessed via ... Compare a string to char *cs2. ... Assign a TSubString substr to TString.
#57. Assign C++ std::string type variable to char type array
I found that the assignment of string to the char array is wrong. I thought that the string has such a copy constructor and can be solved by the following ...
#58. 指標與字串
char *text = "hello";. gcc 沒提出任何警訊,然而 text 儲存了字串常量的位址值,字串常量建立的內容是唯讀的,如果試圖透過 text 改變字元,會發生不可預期的結果:
#59. How can I assign value to a string "char by char" after ...
-value-to-a-string-char-by-char-after-declaration-in-c. ... course ran into the issue of an "incompatible pointer to integer assigning to 'string'" error.
#60. Declaration and initialization of string in c - Log2Base2
Because compiler will automatically assign the null character at the end of string. i)Assigning direct string with size. char country[6]=” ...
#61. String Objects: The string class library
Basic strings (C-strings) are implemented as arrays of type char that are ... To initialize, you can use the assignment operator, and assign a string object ...
#62. String conversion to Char | SAP Community
I have the particular requirement that I want to convert a String type to a char type. Are there any standard functions in ABAP for that?
#63. How to assign a string to a structure variable in C?
#include <string.h> struct name { char *array; }variable; int assign(name *,const char *); int main() { char bla[]="The rain in Spain\n";
#64. String Tutorials & Notes | Python | HackerEarth
You can look into how variables work in Python in the Python variables tutorial. For example, you can assign a character 'a' to a variable ...
#65. Unicode and passing strings — Cython 3.0.0a9 documentation
To convert the byte string back into a C char* , use the opposite assignment: cdef char* other_c_string = py_string # other_c_string is a 0-terminated string ...
#66. Strings Array in C | What is an array of string? - eduCBA
char str_name[8] = “Strings”;. Str_name is the string name and the size defines the length of the string (number of characters). A String can be defined as ...
#67. Chapter 8: Strings
Since C never lets us assign entire arrays, we use the strcpy function to copy one string to another: #include <string.h> char string1[] = "Hello, world!
#68. Stop assigning string literals to char* already! - /dev/krzaq
It is valid in C (C89 through C11), although assigning to a string literal is undefined behaviour there. C++, however, has deprecated this ...
#69. C String – How to Declare Strings in the C Programming ...
Using char , you are able to to represent a single character – out of the 256 that your computer recognises. It is most commonly used to ...
#70. Strings and Character Data in Python
If you were to create a string variable and initialize it to the empty string by assigning it the value 'foo' * -8 , anyone would rightly think you were a ...
#71. How to return a string from a C function - Flavio Copes
If you prefer to assign the result to a variable, you can invoke the function like this: const char* name = myName();.
#72. C++ Character Conversion Functions: char to int, char to string
So the append function will assign those many copies of character to the string as specified in the first argument. assign. Function Prototype: ...
#73. C Programming Course Notes - Character Strings - UIC ...
String literals are stored in C as an array of chars, terminted by a null byte. A null byte is a char having a value of exactly zero, noted as '\0'. Do not ...
#74. String | Android Developers
String (char[] value). Allocates a new String so that it represents the sequence of characters currently contained in the character array ...
#75. String.Split separator won't work with string, only char - UiPath ...
I'm using an assign activity to assign a portion of a long sentence into an array using the split method. I changed the method to accept ...
#76. In Java how to convert String to Char Array? (Two ways)
String crunchifyString = "This is Crunchify";. // toCharArray() converts this string to a new character array. char[] crunchifyCharArray ...
#77. How do I copy char b [] to the content of char * a variable?
These are both ok, and load the address of the string in ROM into the pointer variable. char a [] = "test"; This will create a 5 byte char array ...
#78. How do I create and modify string (character) variables?
In other words, SPSS considers a blank to be a valid value for a string variable. Now let's assign values to gender. We will use the compute and the if commands ...
#79. numpy.chararray — NumPy v1.21 Manual
char module for fast vectorized string operations. Versus a regular NumPy array of type str or unicode , this class adds the following functionality:.
#80. C - Pointers and Strings - C Programming - DYclassroom
In the following code we are assigning the address of the string str to the pointer ptr . char *ptr = str;. We can represent the character pointer variable ...
#81. QString Class | Qt Core 5.15.7 - Qt Documentation
The QString class provides a Unicode character string. ... char (&ch)[N] constructors, and the QString::operator=(const char (&ch)[N]) assignment operator.
#82. How do I write a multi-line string literal in C? - Jim Fisher
What ways do we have to define large string literals in C? Let's take this example: #include <stdio.h> char* my_str = "Here is the first ...
#83. Solved So i am having trouble converting a string into char
i got it to work by assigning char to char array but it doesnt leave much for the setKey function to do, really nothing. i have been playing around with it in ...
#84. String - Kotlin Programming Language
Returns the index of the last character in the char sequence or -1 if it is empty. val CharSequence.lastIndex: Int.
#85. Using PowerShell to split a string into an array - SQLShack
Now, for example, we want to convert the string into the array of the word. Convert Input String in Char Array: ...
#86. Arduino convert string to character array | Circuits4you.com
Example Code for Converting String to Char Array: // Define String str = "This is my string"; // Length (with one extra character for the ...
#87. Why must a pointer to a char array need strcpy to assign ...
When you later assign to a character in the array at now points to, you are assigning a new value to some character in the string literal. That's invoking ...
#88. Replace a Character at a Specific Index in a String in Java
Let's begin with a simple approach, using an array of char. Here, the idea is to convert the String to char[] and then assign the new char at ...
#89. C++ strings: taking string input, pointer to string, passing to ...
We always put string in " ". We can also declare a string variable using characters as follows. char name[ ]= { 'S', 'a' ...
#90. std::string vs C-strings - Embedded Artistry
C-strings are simply implemented as a char array which is terminated by a null ... Assigning values to a std::string is also simple, ...
#91. Python Strings - W3Schools
Strings · Assign String to a Variable · Multiline Strings · Strings are Arrays · Looping Through a String · String Length · Check String · Check if NOT.
#92. Learn C Programming - Strings (char arrays) Part 1 - YouTube
#93. String length - JavaScript - MDN Web Docs
The length property of a String object contains the length of the ... Attempting to assign a value to a string's .length property has no ...
#94. C String char array - Java2s.com
Click the following links for the tutorial for String and char array. ... Assign string value to char pointer and char array · Define and access Character ...
#95. C MCQ Questions and Answers on Strings, Char Arrays and ...
Study C MCQ Questions and Answers on Strings, Character Arrays, String Pointers and Char Pointers. Easily attend technical job interviews after practising ...
#96. String to Char[] - Unity Forum
So, to convert a string into a shuffled char array, just do: Code (CSharp):. Char[] newCharArray = someString.
#97. c++ string详解2 assign - CSDN博客
c. string& assign ( const char* s, size_t n );. 将字符数组或者字符串的首n个字符替换原字符串内容. 举例:. string testassign = "Hello World";.
#98. 2 Character Strings in R - Gaston Sanchez
csv() is file which requires a character string with the pathname of the file. Another example of a basic use of characters is when you assign names to the ...
assign string to char 在 Learn C Programming - Strings (char arrays) Part 1 - YouTube 的美食出口停車場
... <看更多>